Use Terraform to manage Azure Policy
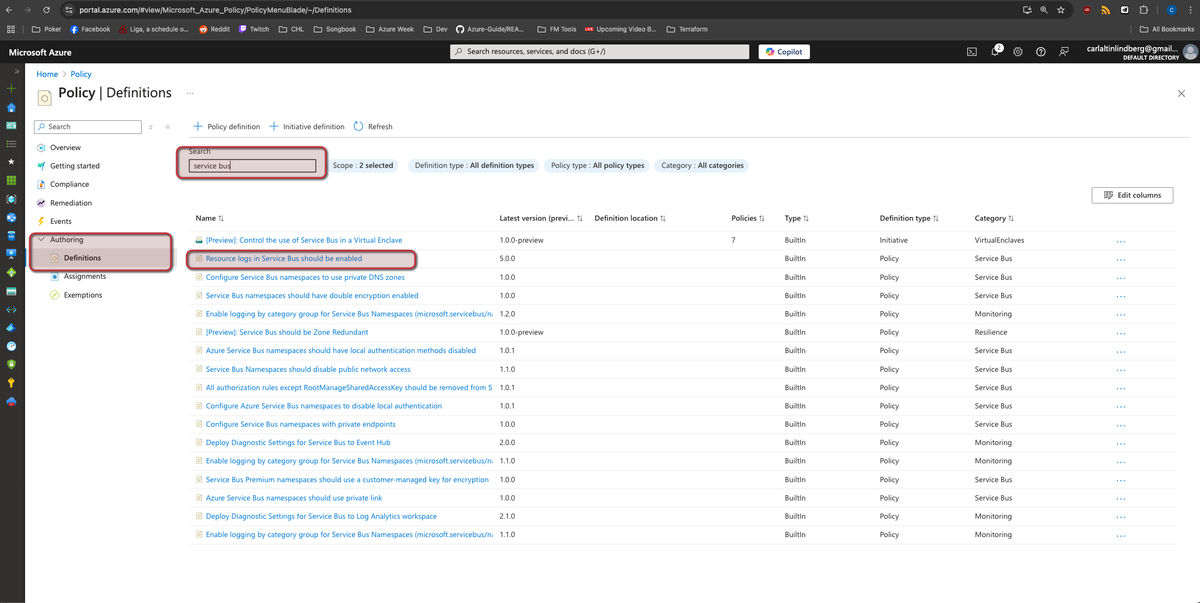
There are several ways you can manage Policy as Code with Microsoft Azure. There are things like Enterprise Policy as Code (EPAC) and Azure Landing Zone Conceptual Architecture (ALZ) (Both Terraform and Azure Bicep options exist). Not to mention the different ALZ accelerators that exist but they are more focused on the portal experience.
All of these are great but can be overwhelming for someone who has never worked with policies before. Not only do you need to know Terraform, you also need to know about certain frameworks and the anatomy of policies in Microsoft Azure.
We will start with some more basic settings. The objective of this post is to teach you the different parts of Azure Policy on a very fundamental level and then author some Terraform Code to provision a Policy Definition and a Policy Assignment.
What is Azure Policy
Azure Policy is an integral part of any cloud administrators toolset for managing a cloud environment. It lets you enforce certain patterns and settings whilst giving you insight into which resources you have that are compliant and non-compliant and let you remediate those.
Policy definition: The rules that are provided for how certain resources and configuration may look and which rules they must follow
Policy definition initiative (sometimes called Set): Many policy definitions combined into one object in the portal which lets you assign several definitions in one fell swoop.
Policy assignment: The second key part. Once you have your rules for how you are allowed to configure your resources (Policy definitions) you will enforce them with policy assignments. There are several ways you can control this with assignments depending on the effect of the policy definition. Some may let you configure resources and become non-compliant whilst some will block updating or creating new resources if they don't follow the rules.
There are more things to Azure Policy, quite a lot more but it will be out of scope for this post. I may cover effect, enforcement mode, remediations and more in other posts if that is of interest.
Author policy with Terraform
Whilst I mentioned EPAC and ALZ previously they often use custom policies in their frameworks but we will do something more simple and use a builtin policy definition which can be found in the Azure Portal.
We will search for Policy and select Definitions and then look for a certain policy for Service Bus
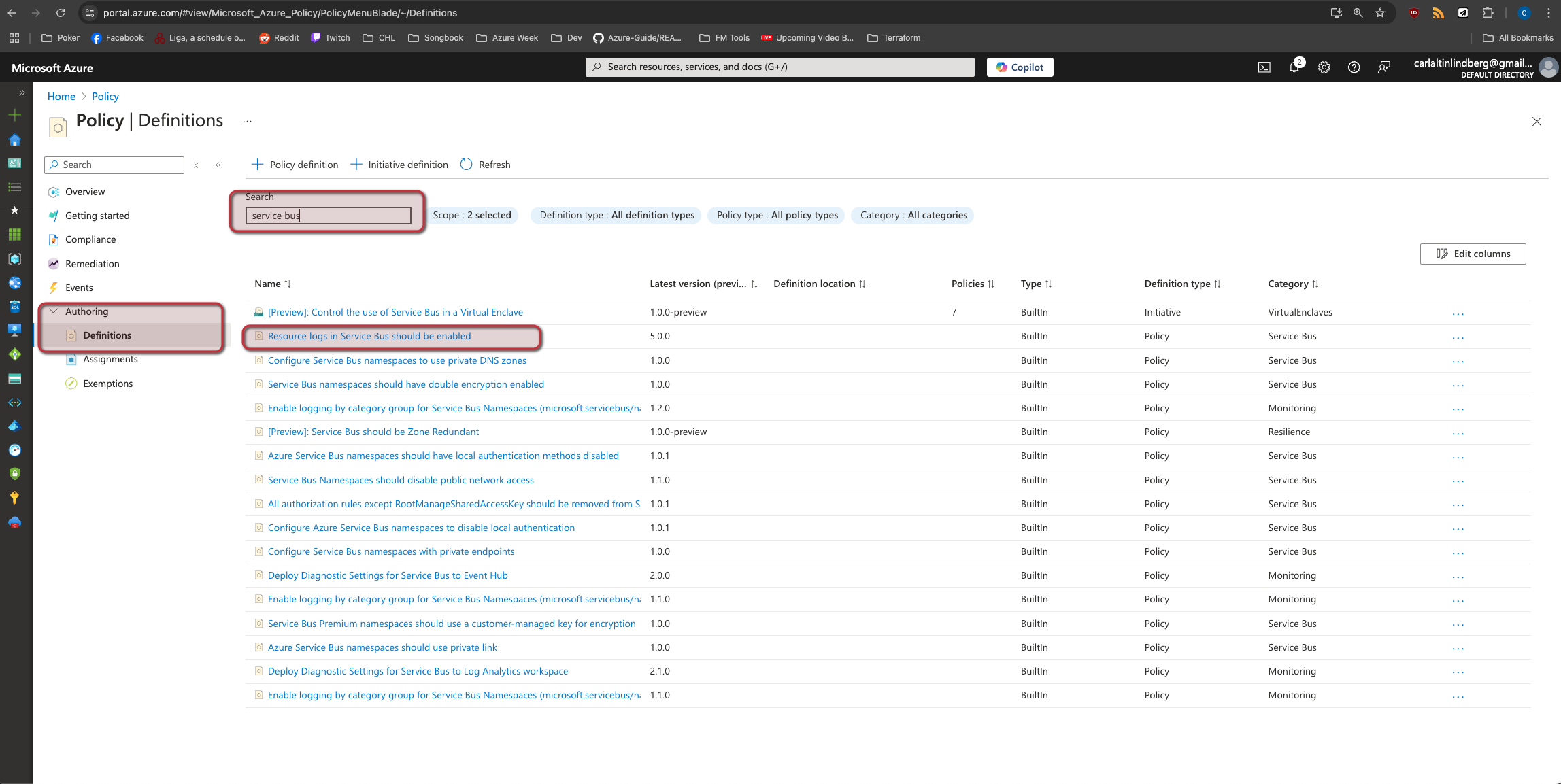
If we select the policy I've marked we will see some key information such as the policy definition ID and the available Parameters we can set which in this case is Effect & requiredRetentionDays with some default values.
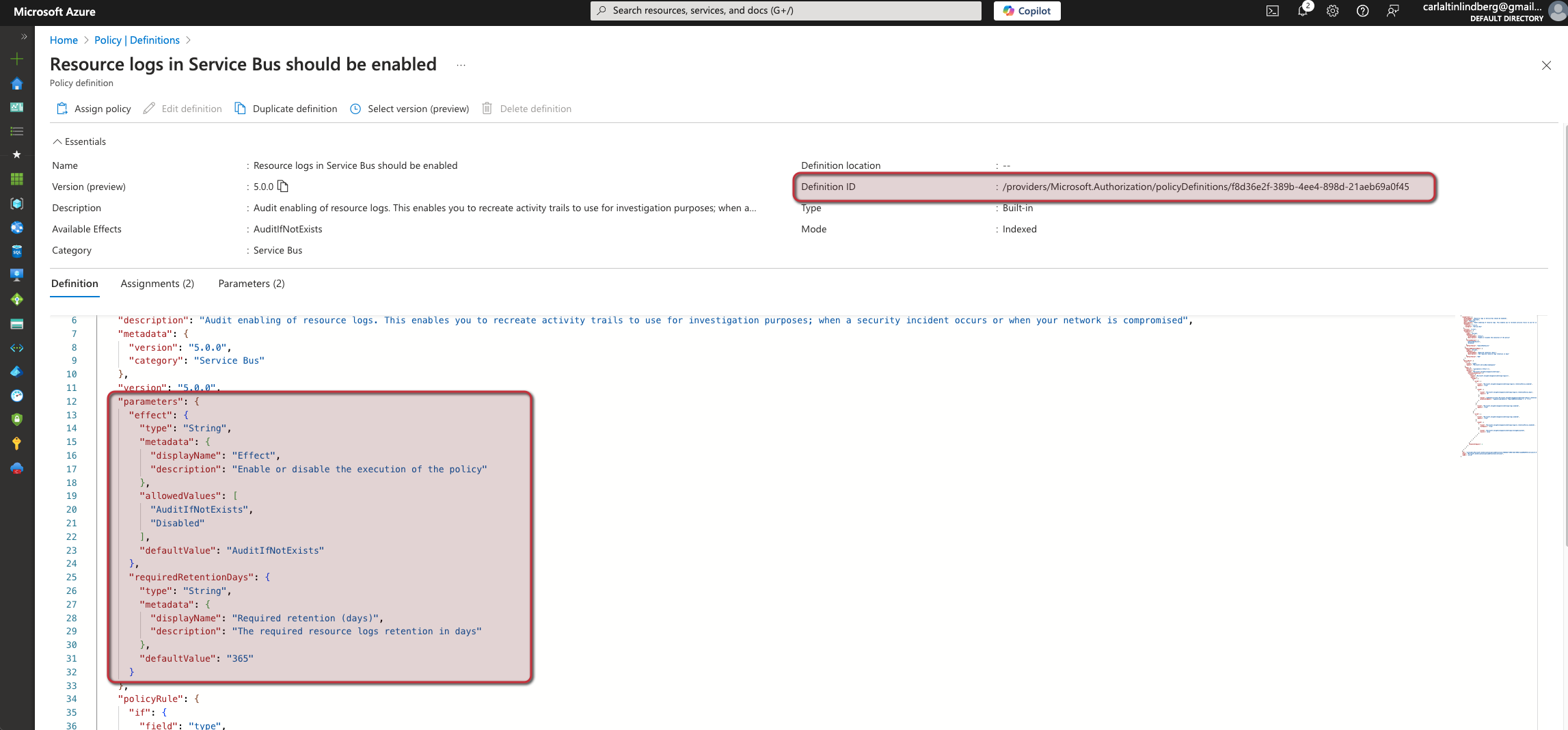
Copy the definition ID, we will need it for later.
Writing the code
I have written the following code which you also can find in the Github Repo HERE
terraform {
required_providers {
azurerm = {
source = "hashicorp/azurerm"
version = "4.6.0"
}
}
}
provider "azurerm" {
features {}
subscription_id = "your-sub-id-here"
}
locals {
subscription_to_assign_policy = "/subscriptions/${var.subscription_id}"
}
data "azurerm_client_config" "current" {}
data "azurerm_policy_definition" "this" {
name = "f8d36e2f-389b-4ee4-898d-21aeb69a0f45" ## ID of the policy definition in the portal
}
resource "azurerm_subscription_policy_assignment" "this" {
name = data.azurerm_policy_definition.this.display_name
policy_definition_id = data.azurerm_policy_definition.this.id
subscription_id = local.subscription_to_assign_policy
parameters = jsonencode({
"Effect" = {
"value" = "AuditIfNotExists"
}
"requiredRetentionDays" = {
"value" = "90"
}
})
}
Here we have set the two parameters to AuditIfNotExists so if resource log configuration is missing on the Service Bus resource it will be marked as non-compliant and requiredRententionDays to 90 days instead of the default 365.
Running terraform apply
and supplying my subscription ID:
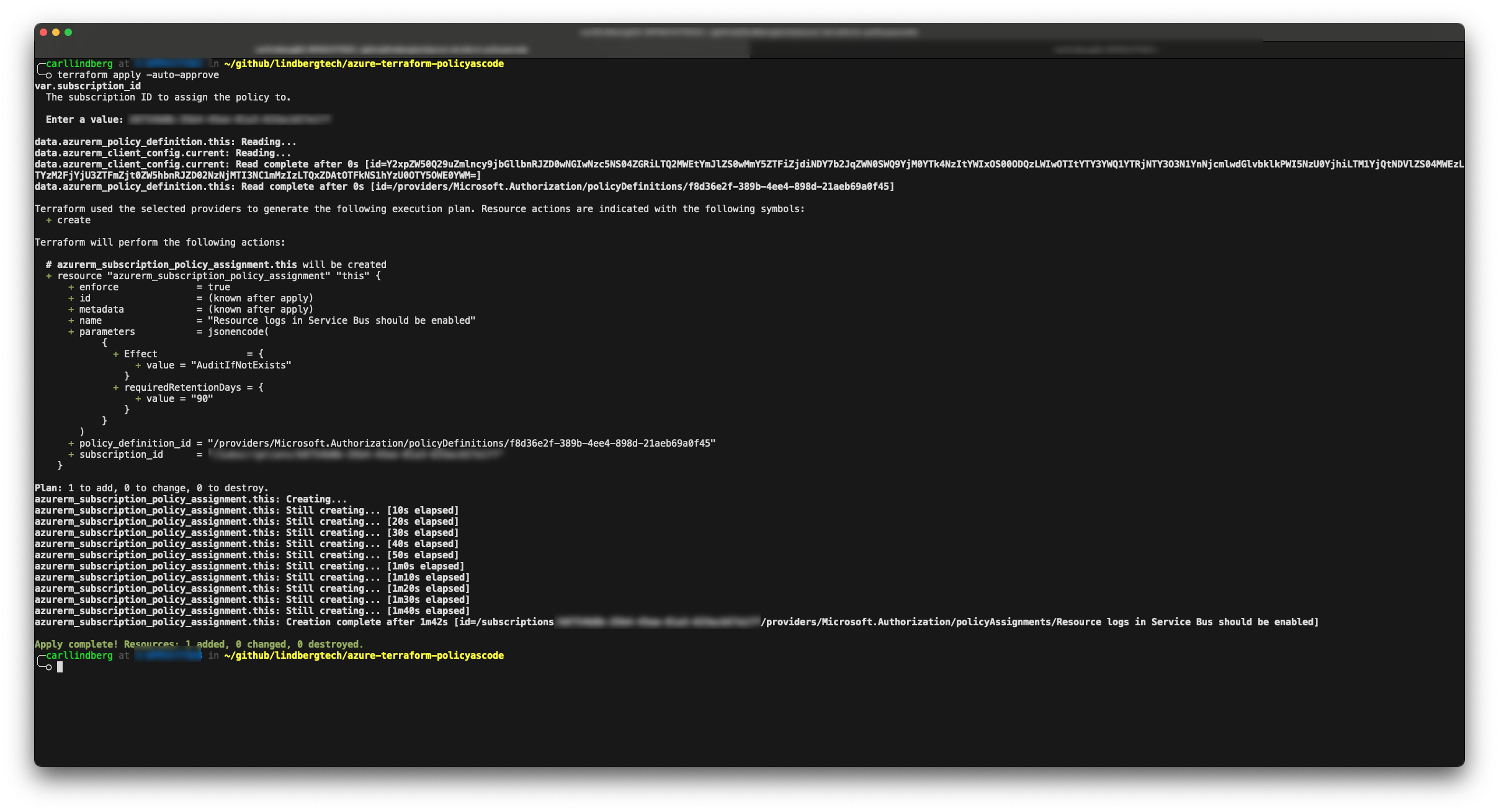
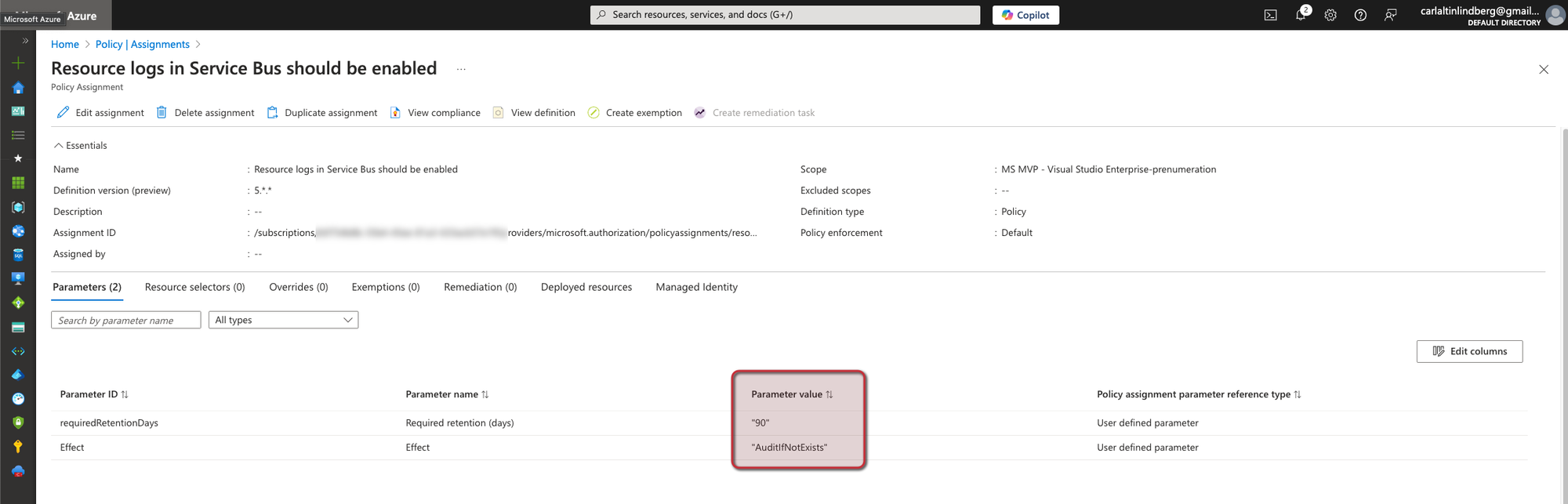
Conclusion
We were with quite a small effort able to create a policy assignment, set some custom values and apply it with Terraform. The benefit with this is that:
- All settings are documented in code
- Any changes will be reviewed by a peer if 4-eyes principles with PRs are applied
- Any changes will be documented in source control with a commit message as to why the change happened
Hope you enjoyed!
References
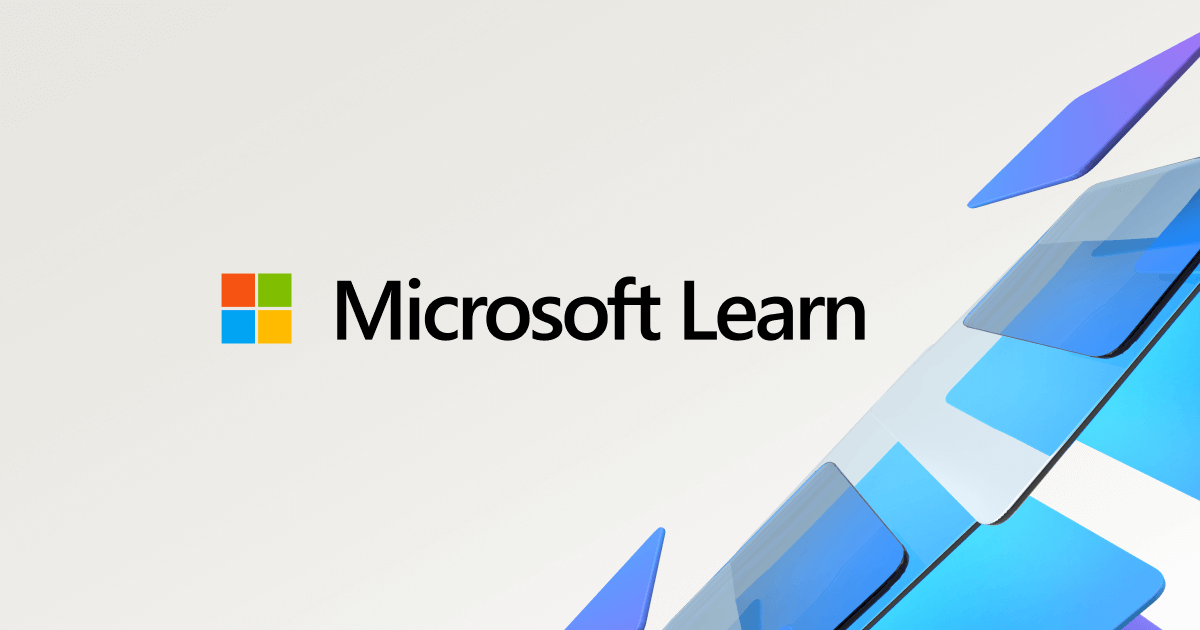
About me
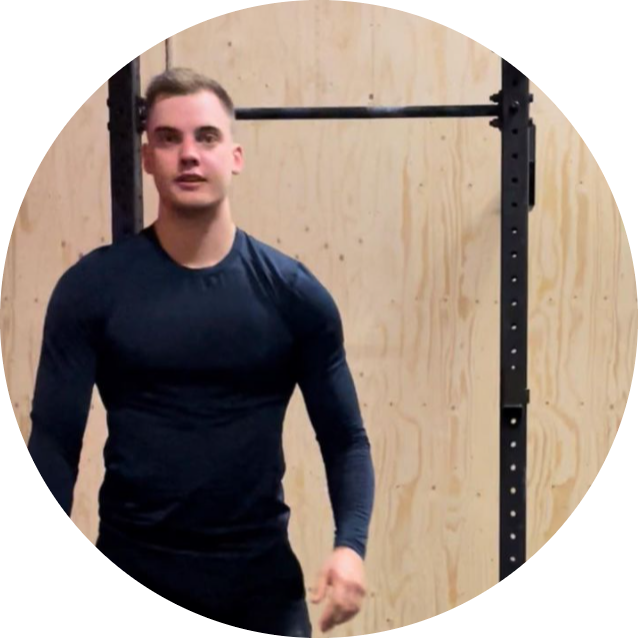